C/C++ PROGRAMMING
This course is specially designed for University and College students to learn C / C++ from basic to advance level. C++ is the object-oriented version of C that has been widely used to develop enterprise and commercial applications. Created by Bjarne Stroustrup, C++ became popular because it combined traditional C programming with object-oriented programming (OOP) features. If you are curious to start your career in Software Development and Software Engineering then this course is the best starting point for you.
Course Contents
- Introduction to C++ Programming
- Flow Chart
- Basic Input and Output
- Flow Control
- Conditional Statements
- Loops
- Arrays
- Functions
- Pointers
- Structures
- Classes
- Objects
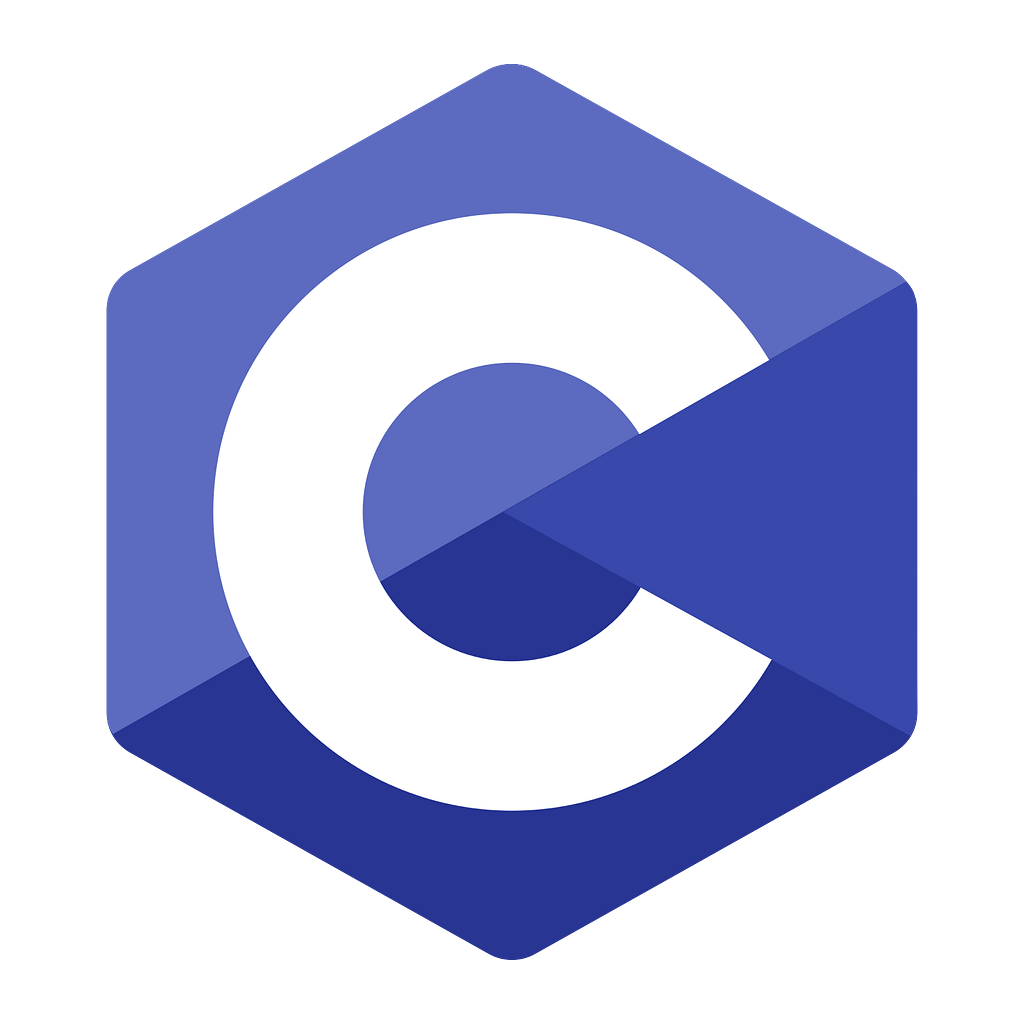
Course Outline for C and C++ Compiled By Sardar Azeem
Week 1: Introduction to C Programming
- Day 1: Overview of C and C++, Compiler Installation (GCC), and IDEs
- Intro to programming, what is C, and installing necessary tools (like Code::Blocks, Visual Studio, or GCC).
- Day 2: Basic Syntax in C
- Data types, variables, keywords, constants, basic input/output (printf, scanf)
- Day 3: Operators and Expressions
- Arithmetic, relational, logical, bitwise, and assignment operators
- Day 4: Control Structures I
- If-else, switch-case, nested if, loops (for, while, do-while)
- Day 5: Functions and Recursion
- Writing functions, call by value, call by reference, recursion basics
- Day 6: Arrays and Strings in C
- One-dimensional and two-dimensional arrays, string manipulation (strlen, strcpy, strcat, etc.)
- Day 7: Weekend Review
- Practice exercises on control structures, functions, arrays
Week 2: Advanced C Programming
- Day 8: Pointers
- Introduction to pointers, pointer arithmetic, pointers with arrays and functions
- Day 9: Dynamic Memory Allocation
- malloc, calloc, free, realloc
- Day 10: Structures and Unions
- Defining and using structures, nested structures, unions, and bit-fields
- Day 11: File Handling
- File I/O operations (fopen, fclose, fread, fwrite, fscanf, fprintf)
- Day 12: Preprocessor Directives and Macros
- #define, #include, macros, conditional compilation
- Day 13: Review and C Project Assignment
- Practice questions, debugging exercises, and a small C-based project
- Day 14: Weekend Project
- Work on the project assigned (such as a file management system or a mini calculator)
Week 3: Transition to C++ Programming
- Day 15: Overview of C++
- Introduction to Object-Oriented Programming (OOP), comparison of C and C++, basic syntax in C++
- Day 16: Functions in C++
- Function overloading, inline functions, default arguments, pass by reference
- Day 17: Introduction to Classes and Objects
- Defining classes, creating objects, member functions, accessing data members
- Day 18: Constructors and Destructors
- Constructor types, overloading constructors, destructors
- Day 19: Static Members and Friend Functions
- Static variables, static member functions, friend functions and classes
- Day 20: Review and Coding Practice
- Solve problems related to functions, classes, and constructors
- Day 21: Weekend Review
- Deep dive into C++ concepts practiced during the week, working on small projects
Week 4: Advanced C++ OOP Concepts
- Day 22: Operator Overloading
- Overloading unary and binary operators, friend operator functions
- Day 23: Inheritance I
- Single, multilevel inheritance, constructor invocation in inheritance
- Day 24: Inheritance II
- Multiple and hierarchical inheritance, virtual base classes
- Day 25: Polymorphism I
- Function overriding, virtual functions, runtime polymorphism
- Day 26: Polymorphism II
- Abstract classes, pure virtual functions
- Day 27: Templates in C++
- Function templates, class templates, specialization
- Day 28: Weekend Project
- Develop a class-based C++ project such as a simple banking system or employee management system
Week 5: Advanced C++ Features
- Day 29: Exception Handling
- Try, catch, throw, nested try-catch blocks, exception hierarchy
- Day 30: File Handling in C++
- File streams, reading from and writing to files, file pointers
- Day 31: Standard Template Library (STL) I
- Introduction to STL, vectors, lists, and iterators
- Day 32: Standard Template Library (STL) II
- Sets, maps, algorithms (sort, find, etc.)
- Day 33: Namespaces and Dynamic Memory in C++
- Using namespaces, memory management in C++ (new, delete)
- Day 34: Review and Practice
- Practice exercises on inheritance, polymorphism, templates, and STL
- Day 35: Weekend Review
- Complete exercises on exception handling, STL, and file handling
Week 6: Final Projects and Applications
- Day 36: Introduction to Algorithms and Data Structures in C/C++
- Basic sorting (bubble, selection, quick sort), searching (linear, binary search)
- Day 37: Linked Lists and Stacks
- Singly and doubly linked lists, stack implementation using arrays and linked lists
- Day 38: Queues and Trees
- Circular queue, priority queue, introduction to trees, binary trees, traversals
- Day 39: Final Project Discussion
- Discuss final project requirements, outline structure and features
- Day 40: Start Final Project
- Work on a medium-sized project (such as a mini-compiler, a library system, or a basic game)
- Day 41: Continue Working on Final Project
- More coding and implementation for the final project
- Day 42: Project Submission and Review
- Final project submission, code review, discussion on optimizations and improvements
Week 7-8: Additional Topics and Practice (Optional)
For these two weeks, you can focus on any of the following topics or spend time deepening concepts:
- Advanced Data Structures (Heaps, Graphs)
- Multithreading in C++
- More practice with real-world projects
- Deep dive into algorithms for competitive programming
- Revisiting complex C++ OOP topics (virtual destructors, multiple inheritance pitfalls, etc.)
This course provides enough time to grasp fundamental to advanced concepts in C/C++ and complete meaningful projects that solidify understanding.